Procedural Mesh with marching squares
Description
A continuation of Sebastian Lague's
procedural planet generation tutorial
. In this project, I learned how to create meshes in code by making an ocean mesh that follows the contour of the planet's
land. My solution was to implement the marching squares algorithm.
You divide the mesh into squares and you give the corners a value
of how close it is to the ocean level. Then, you get the correct configuration of the mesh in that cell from the lookup table that has
all the possible ways a cell can look like.
The main challenge was understanding how the generation of meshes works, as well as finding the right solution. I randomly
stumbled upon the square marching algorithm and realized that I could use it for this project. Implementing the algorithm was hard, but I had
a lot of fun doing it.
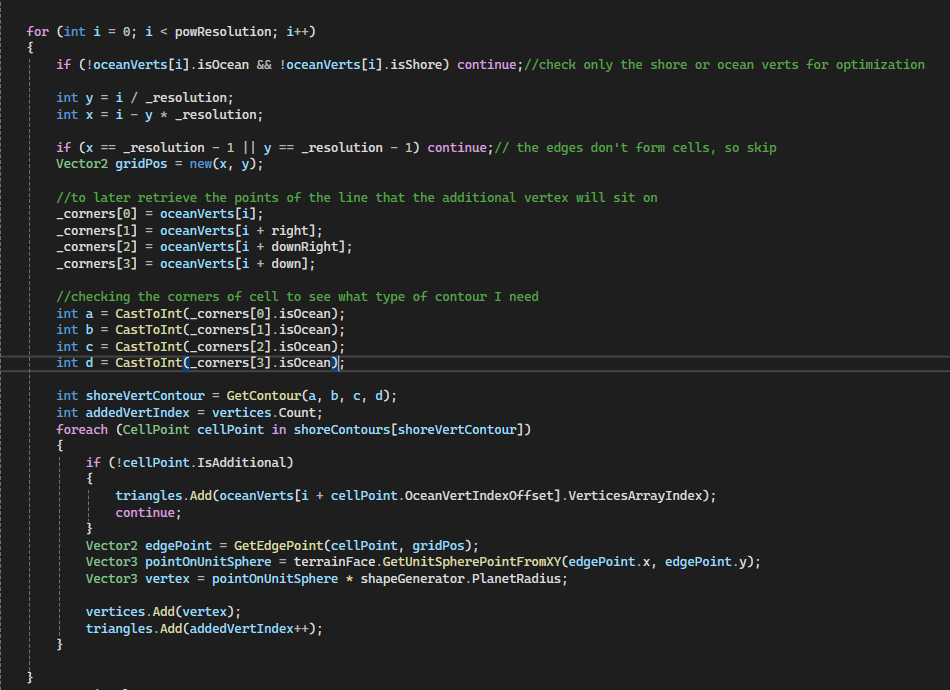